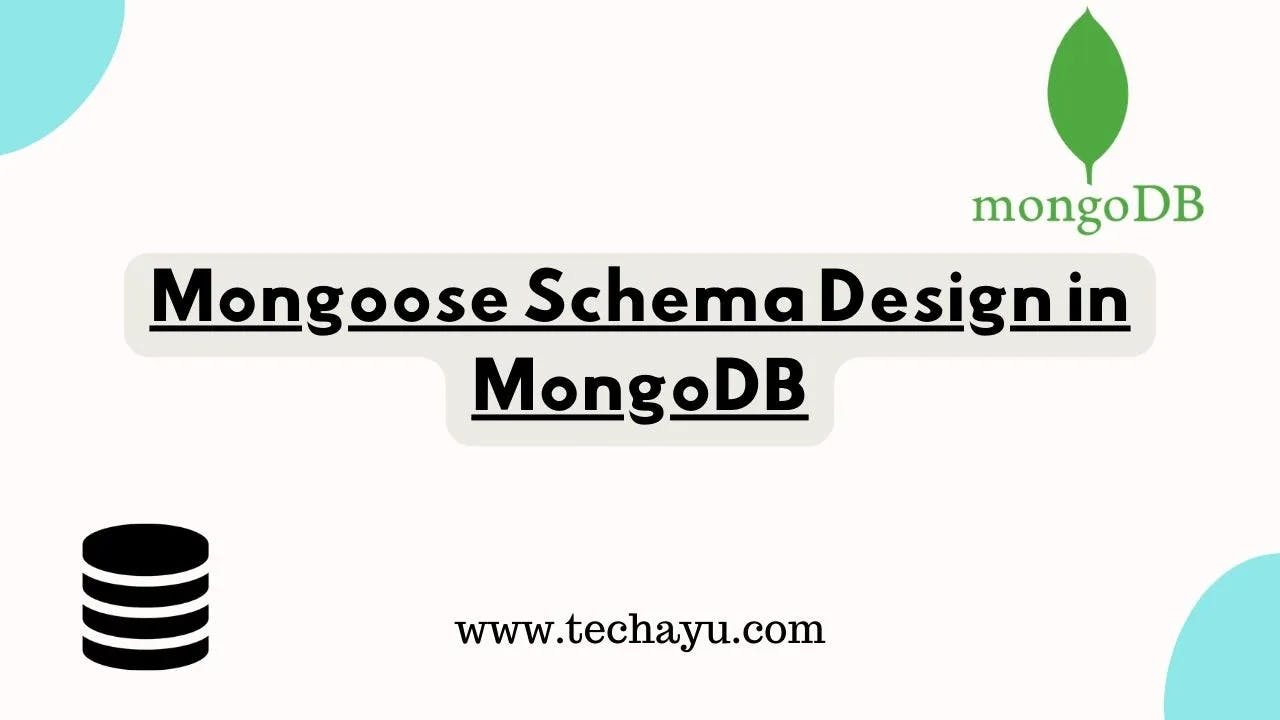
How to Use Mongoose Schema Design in MongoDB
Mongoose, a popular ODM (Object Data Modeling) library for MongoDB and Node.js, adds an extra layer of abstraction for schema management and validation. In this guide, we’ll explore the intricacies of Mongoose Schema Design to elevate your MongoDB data modeling.
Understanding Mongoose Schema
Mongoose simplifies MongoDB interactions by providing a structured schema, middleware, and validation for data. The first step is to understand the fundamentals of Mongoose schema, which includes defining the structure of documents and their data types.
Step 1: Install and Set Up Mongoose
Begin by installing Mongoose in your Node.js project using npm or yarn:
npm install mongoose
# or
yarn add mongoose
After installation, set up Mongoose in your application by connecting to your MongoDB database:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/your-database', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
Step 2: Define a Mongoose Schema
Create a Mongoose schema by defining the structure of your documents using Mongoose data types. Specify fields, types, and optional validation:
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
username: { type: String, required: true },
email: { type: String, required: true, unique: true },
age: { type: Number, min: 18 },
});
const User = mongoose.model('User', userSchema);
Step 3: Perform CRUD Operations
Utilize your Mongoose schema to perform CRUD (Create, Read, Update, Delete) operations. Create new documents, retrieve data, update records, and delete entries seamlessly:
// Create
const newUser = new User({ username: 'john_doe', email: 'john@example.com', age: 25 });
newUser.save();
// Read
User.find({ age: { $gte: 21 } }, (err, users) => {
console.log(users);
});
// Update
User.updateOne({ username: 'john_doe' }, { age: 26 }, (err, result) => {
console.log(result);
});
// Delete
User.deleteOne({ username: 'john_doe' }, (err) => {
if (!err) console.log('User deleted successfully');
});
Step 4: Implement Middleware
Mongoose middleware allows you to execute functions before or after specific operations. Implement middleware to add custom logic, validations, or modifications to your data:
userSchema.pre('save', function (next) {
// Custom logic before saving
console.log('Saving user...');
next();
});
Step 5: Leverage Mongoose Plugins
Extend the functionality of your Mongoose schema by using plugins. Plugins are reusable pieces of functionality that can be applied to multiple schemas:
const timestampPlugin = (schema, options) => {
schema.add({ createdAt: { type: Date, default: Date.now } });
schema.add({ updatedAt: { type: Date, default: Date.now } });
schema.pre('updateOne', function (next) {
this.update({}, { $set: { updatedAt: new Date() } });
next();
});
};
userSchema.plugin(timestampPlugin);
Conclusion: Mastering Mongoose Schema Design
Mongoose Schema Design provides a powerful framework for structuring and managing MongoDB data in Node.js applications. By following these steps, you can harness the full potential of Mongoose for robust data modeling, validation, and middleware customization.
Delve into the world of Mongoose Schema Design, embrace its flexibility, and elevate your MongoDB interactions to new heights. Happy coding!