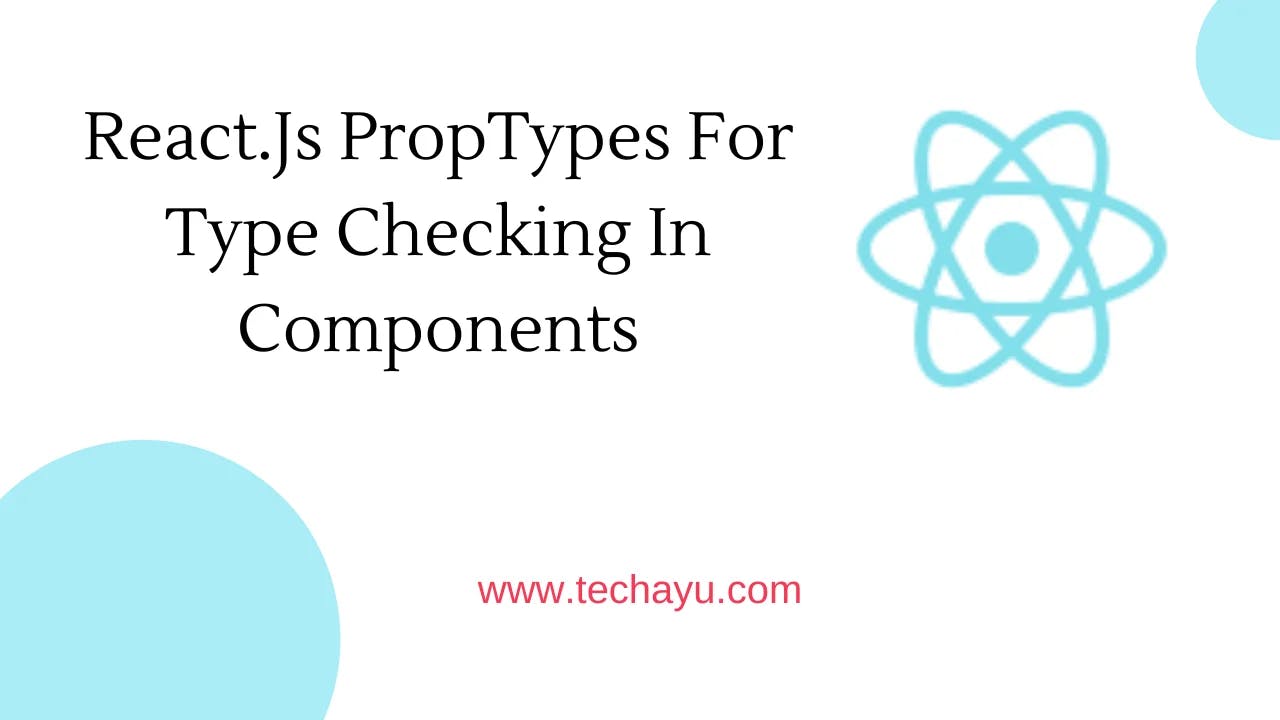
How To Use React.Js PropTypes
Ensuring the correct types of data flow through your React.js components is crucial for preventing bugs and maintaining a stable codebase. React.js provides a powerful solution to this challenge through the use of PropTypes. In this guide, we’ll explore how to leverage React.js PropTypes for effective type checking in your components.
Understanding React.js PropTypes
PropTypes is a runtime type-checking feature in React. It helps you define the expected data types of your component’s props, making it easier to catch and address potential issues during development.
Step 1: Installing Prop-Types Library
Start by installing the prop-types
library using npm or yarn:
npm install prop-types
# or
yarn add prop-types
Step 2: Importing PropTypes
In your React component file, import PropTypes from the prop-types
library:
1import PropTypes from 'prop-types';
import PropTypes from 'prop-types';
Step 3: Defining PropTypes
Within your component, define PropTypes for the expected data types of your props:
import React from 'react';
import PropTypes from 'prop-types';
function MyComponent({ name, age, isStudent }) {
// Component logic
return (
// Component rendering
);
}
MyComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
isStudent: PropTypes.bool.isRequired,
};
export default MyComponent;
Step 4: Utilizing PropTypes Features
Explore additional features of PropTypes, such as isRequired
to mark a prop as mandatory, and shape
to define an object with specific prop types.
MyComponent.propTypes = {
person: PropTypes.shape({
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
}).isRequired,
hobbies: PropTypes.arrayOf(PropTypes.string),
};
Step 5: Detecting Type Errors in Development
React.js will now throw warnings in the console if a component receives props of incorrect types, providing valuable insights during development.
Conclusion: Strengthen Your React Components with PropTypes
By implementing PropTypes in your React.js components, you establish a robust system for type checking, enhancing code reliability and maintainability. Embrace this feature to catch errors early in the development process, allowing for smoother collaboration and a more stable application.
Experiment with different PropTypes configurations based on your specific project requirements, and make the most of this powerful tool in your React.js development journey. Happy coding!