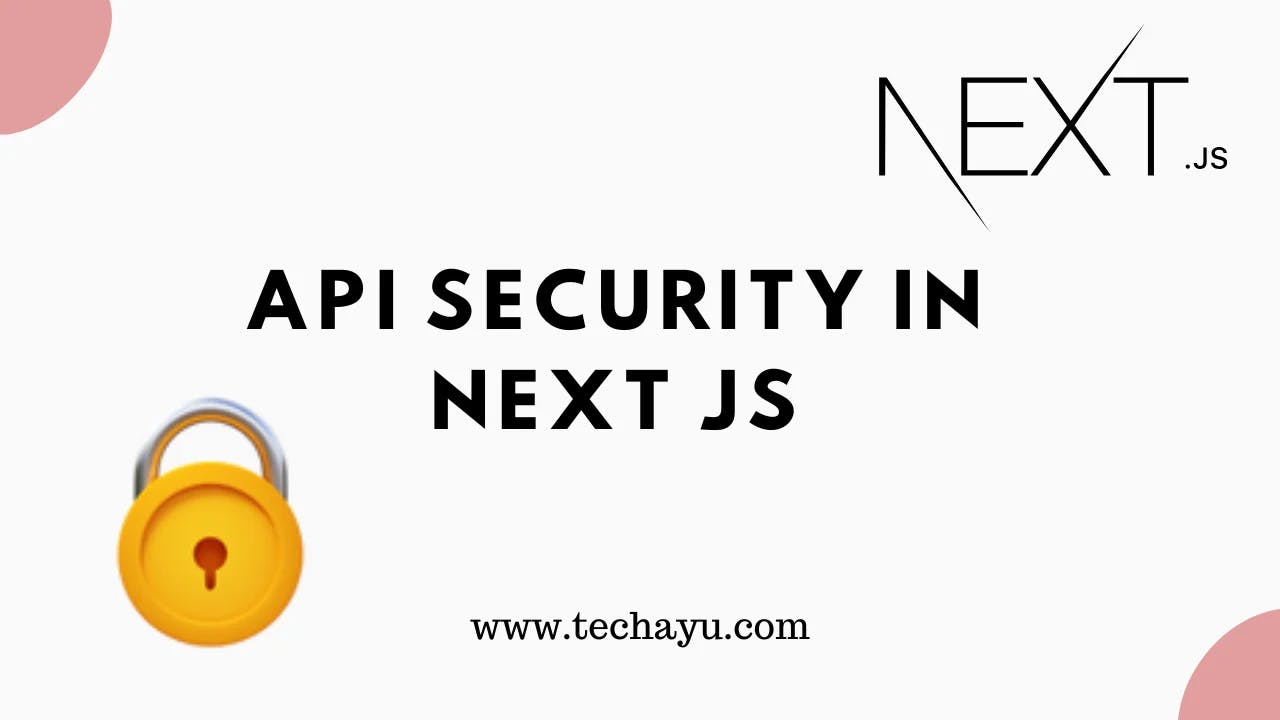
How To Secure APIs In Next JS
Introduction
In the realm of web development, ensuring the security of APIs (Application Programming Interfaces) is paramount, particularly in Next.js applications, where APIs play a crucial role in connecting front-end interfaces with back-end functionalities. This article delves into the significance of API security within Next.js projects and explores strategies to fortify them against potential threats.
Importance of API Security in Next.js
Next.js, a popular React framework, empowers developers to build powerful, dynamic web applications with ease. However, as these applications rely heavily on APIs to fetch and manipulate data, any vulnerability within these APIs could jeopardize the entire system. Hence, prioritizing API security is imperative to safeguard sensitive information and maintain user trust.
Risks of Insecure APIs
Unsecured APIs expose Next.js applications to various risks, including data breaches, unauthorized access, and malicious attacks. Hackers may exploit vulnerabilities within unprotected APIs to intercept data transmissions, inject malicious code, or perform unauthorized actions, compromising the integrity and confidentiality of the application’s data.
Authentication and Authorization
Authentication and authorization mechanisms play a pivotal role in ensuring the integrity and confidentiality of data transmitted through APIs.
Authentication and Authorization Concepts
Authentication verifies the identity of users accessing the system, while authorization determines the actions they’re allowed to perform. In Next.js applications, implementing robust authentication and authorization mechanisms is crucial to prevent unauthorized access to sensitive resources.
Implementing User Authentication with NextAuth.js
NextAuth.js simplifies the process of implementing authentication in Next.js applications. By integrating NextAuth.js, developers can enable features like email/password authentication, social authentication (e.g., Google, Facebook), and multi-factor authentication with minimal configuration.
// Example of setting up authentication with NextAuth.js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
// Configure one or more authentication providers
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
// Add more providers as needed
],
// Additional NextAuth.js configurations
});
Securing Client-side Rendered Pages
Client-side rendered pages in Next.js applications can be secured using the useSession
React Hook provided by NextAuth.js. This Hook allows developers to retrieve the user’s session information and restrict access to authenticated users only.
// Example of securing client-side rendered pages with useSession
import { useSession } from 'next-auth/react';
function ProfilePage() {
const { data: session, status } = useSession();
if (status === 'loading') {
return <div>Loading...</div>;
}
if (!session) {
// Redirect to login page if user is not authenticated
return <LoginPage />;
}
// Render profile page for authenticated users
return (
<div>
<h1>Welcome, {session.user.name}!</h1>
<p>Email: {session.user.email}</p>
{/* Add more profile information */}
</div>
);
}
Protecting Server-side Rendered Pages
Server-side rendered pages require additional security measures to validate user sessions. NextAuth.js provides the getServerSession
method, which facilitates server-side session management and ensures that only authenticated users can access protected routes.
// Example of protecting server-side rendered pages with getServerSession
import { getServerSession } from 'next-auth/react';
export async function getServerSideProps(context) {
const session = await getServerSession(context);
if (!session) {
// Redirect to login page if user is not authenticated
return {
redirect: {
destination: '/login',
permanent: false,
},
};
}
// Fetch data for authenticated users
const userData = await fetchData();
return {
props: {
userData,
},
};
}
Securing API Routes
Secure API routes are essential for safeguarding sensitive data and preventing unauthorized access within Next.js applications.
Overview of Secure API Routes in Next.js
Next.js offers built-in functionality for creating secure API routes using the api
directory. These routes can be protected using various authentication mechanisms to restrict access to authorized users only.
Using getSession()
for Protection
The getSession()
function provided by NextAuth.js allows developers to retrieve the user’s session information within API routes. By validating the user’s session, developers can enforce authentication requirements for accessing protected API endpoints.
// Example of protecting API routes with getSession
import { getSession } from 'next-auth/react';
export default async function handler(req, res) {
const session = await getSession({ req });
if (!session) {
// Return 401 Unauthorized if user is not authenticated
res.status(401).json({ error: 'Unauthorized' });
return;
}
// Handle authenticated requests
res.status(200).json({ message: 'Authenticated request' });
}
Leveraging JSON Web Tokens (JWT)
JSON Web Tokens (JWT) provide a secure method for transmitting authentication data between the client and server. Next.js applications can utilize JWTs to verify the authenticity of incoming requests and enforce access control policies within API routes.
// Example of JWT validation in API routes
import jwt from 'jsonwebtoken';
export default async function handler(req, res) {
// Retrieve JWT token from request headers or cookies
const token = req.headers.authorization?.replace('Bearer ', '');
try {
// Verify JWT token
const decodedToken = jwt.verify(token, process.env.JWT_SECRET);
// Handle authenticated requests
res.status(200).json({ user: decodedToken });
} catch (error) {
// Return 401 Unauthorized for invalid tokens
res.status(401).json({ error: 'Unauthorized' });
}
}
Creating Middleware for Security
Middleware functions offer a flexible approach to implementing security checks within API routes. By creating custom middleware, developers can enforce authentication, authorization, and other security policies to protect sensitive endpoints from unauthorized access.
// Example of creating custom middleware for authentication
export function authenticateMiddleware(req, res, next) {
const session = getSessionFromRequest(req);
if (!session) {
// Return 401 Unauthorized if user is not authenticated
res.status(401).json({ error: 'Unauthorized' });
return;
}
// User is authenticated, proceed to next middleware or route handler
next();
}
Handling Sensitive Data
Effectively managing sensitive data is crucial for maintaining the security and integrity of Next.js applications.
Best Practices for Managing Sensitive Keys
Sensitive API keys, such as access tokens and secret keys, should be handled with care to prevent exposure to unauthorized parties. Utilizing secure storage mechanisms, such as environment variables or secret management services, can mitigate the risk of key compromise.
Securely Storing Environment Variables
Next.js applications can securely store sensitive information, such as API keys and database credentials, using environment variables. By storing sensitive data outside of the application codebase, developers can prevent accidental exposure and unauthorized access to critical resources.
Example of Moving API Calls
To enhance security and maintain code modularity, developers can encapsulate API calls within separate handler functions. By isolating API logic from the main application code, developers can improve maintainability and facilitate easier integration of security measures, such as input validation and error handling.
// Example of moving API calls to a separate handler function
import axios from 'axios';
export async function fetchData() {
try {
const response = await axios.get('/api/data');
return response.data;
} catch (error) {
console.error('Failed to fetch data:', error);
throw error;
}
}
Conclusion
In conclusion, securing APIs in Next.js applications is paramount to protect against potential threats and ensure the confidentiality and integrity of sensitive data. By implementing robust authentication, authorization, and data protection mechanisms, developers can fortify their applications against malicious attacks and safeguard user information.
FAQs (Frequently Asked Questions)
1. Why is API security important in Next.js?
API security is crucial in Next.js to protect against data breaches, unauthorized access, and malicious attacks that could compromise sensitive information.
2. How can NextAuth.js enhance API security?
NextAuth.js simplifies the implementation of authentication in Next.js applications, providing features like email/password authentication, social authentication, and multi-factor authentication.
3. What are the benefits of using JWTs in Next.js applications?
JSON Web Tokens (JWTs) provide a secure method for transmitting authentication data between the client and server, enabling robust access control and data integrity mechanisms.
4. How should developers handle sensitive API keys in Next.js?
Developers should follow best practices for managing sensitive keys, such as storing them securely using environment variables or secret management services to prevent exposure to unauthorized parties.
5. Why is it important to secure API routes in Next.js?
Securing API routes is essential to prevent unauthorized access to sensitive data and ensure compliance with privacy and security regulations.