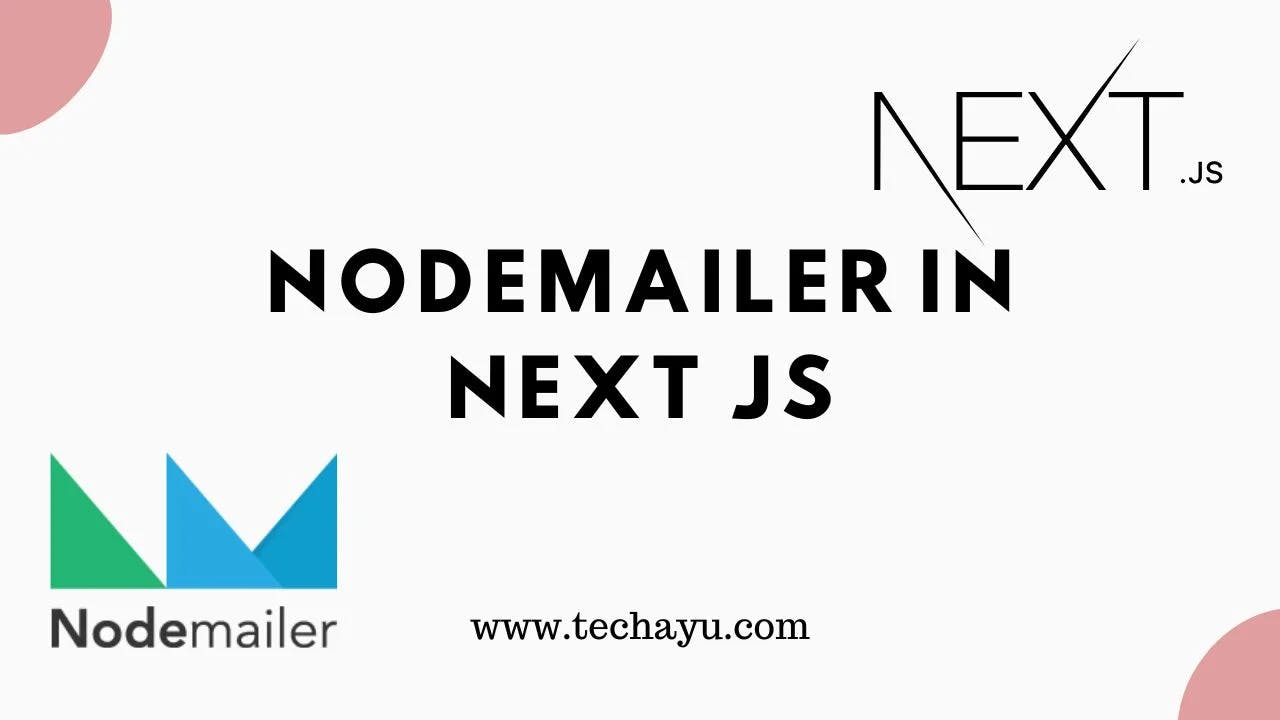
How to use Nodemailer in Next JS
Harnessing the Power of Nodemailer in Next.js: A Step-by-Step Guide
Are you looking to supercharge your Next.js application with the ability to send emails seamlessly? Look no further! In this tutorial, we’ll explore how to integrate Nodemailer, a popular Node.js module, into your Next.js project. By the end of this guide, you’ll have a robust contact form that not only captures user inquiries but also sends informative emails to both you and the user.
Why Nodemailer?
Nodemailer simplifies the process of sending emails using Node.js. Its versatility and ease of use make it an ideal choice for applications built with frameworks like Next.js. In this guide, we’ll focus on incorporating Nodemailer into a contact form within a Next.js project.
The Power of Nodemailer in Next.js
Imagine a scenario where you not only capture user inquiries but also engage with them through personalized email responses. That’s precisely what we aim to achieve with Nodemailer in Next.js. Let’s break down the steps to harness this power for your application.
Step 1: Installation and Configuration
First things first, ensure you have Nodemailer installed in your Next.js project. Use the command mentioned above for a smooth installation process.
npm install nodemailer
Step 2: Setting Up Nodemailer Transporter
The createTransport
function sets up the Nodemailer transporter. In our example, we’ve chosen Gmail as the email service. However, you can configure it to work with other services as per your preference.
const transporter = nodemailer.createTransport({
service: "Gmail",
auth: {
user: process.env.EMAIL,
pass: process.env.SECRET_KEY,
},
});
Step 3: Crafting Informative Emails
The mailOptions
and mailOptions2
objects define the content of the emails. The first email includes the user’s details and is sent to your specified email address. The second email serves as a thank-you note and is sent to the user.
Step 4: Sending Emails
The magic happens with the sendMail
function. By invoking this function on the transporter, you initiate the process of sending the emails. The asynchronous nature ensures a smooth flow, and errors are handled gracefully.
await transporter.sendMail(mailOptions);
await transporter.sendMail(mailOptions2);
Step 5: Error Handling
In the event of any errors during the email send process, the code catches them, allowing you to respond appropriately.
try {
// Sending emails
} catch (error) {
// Handling errors
}
Conclusion
By integrating Nodemailer into your Next.js project, you’ve unlocked a new level of interaction with your users. The ability to seamlessly send personalized emails not only keeps you informed but also enhances the user experience. Feel free to customize the code further to align it with your specific use case.
Complete Code
For your convenience, here’s the complete code you can use in your Next.js project:
//pages/api/contact.js
import nodemailer from "nodemailer";
export default async function handler(req, res) {
if (req.method === "POST") {
const { fullname, email, phone, msg } = req.body;
// Create a Nodemailer transporter
const transporter = nodemailer.createTransport({
service: "Gmail",
auth: {
user: process.env.EMAIL,
pass: process.env.SECRET_KEY,
},
});
// Create a Nodemailer email message
const mailOptions = {
from: process.env.EMAIL,
to: process.env.EMAIL,
subject: "Take advantage of the services",
html: `<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Table Email Template</title>
<style type="text/css">
/* Add your custom styles here */
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
padding: 50px;
}
h1 {
font-size: 36px;
color: #333;
text-align: center;
margin-bottom: 50px;
}
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 50px;
}
th,
td {
padding: 10px 20px;
text-align: left;
border-bottom: 1px solid #ddd;
}
th {
background-color: #333;
color: #fff;
font-size: 18px;
}
td {
font-size: 16px;
color: #555;
}
</style>
</head>
<body>
<h1>Your Recent Details</h1>
<table>
<thead>
<tr>
<th>FUll Name</th>
<th>Email</th>
<th>Phone</th>
<th>Massege</th>
</tr>
</thead>
<tbody>
<tr>
<td>${fullname}</td>
<td>${email}</td>
<td>${phone}</td>
<td>${msg}</td>
</tr>
</tbody>
</table>
</body>
</html>
`,
};
// Create a Nodemailer email message for the second email
const mailOptions2 = {
from: process.env.EMAIL,
to: email,
subject: "Take advantage of the services",
html: `<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Thank You Email Template</title>
<style type="text/css">
/* Add your custom styles here */
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
padding: 50px;
text-align: center;
}
h1 {
font-size: 36px;
color: #333;
margin-bottom: 20px;
}
p {
font-size: 18px;
color: #555;
line-height: 1.5;
margin-bottom: 50px;
}
.thank-you-icon {
width: 100px;
height: 100px;
margin: 0 auto;
background-image: url(https://via.placeholder.com/100x100);
background-repeat: no-repeat;
background-position: center;
background-size: contain;
}
.btn {
display: inline-block;
padding: 10px 20px;
font-size: 18px;
color: #fff;
background-color: #333;
border-radius: 20px;
text-decoration: none;
}
</style>
</head>
<body>
<div class="thank-you-icon"></div>
<h1>Thank You for details!</h1>
<a href="theclockmedia.tech" class="btn">Learn More</a>
</body>
</html>
`,
};
try {
// Send the first email
await transporter.sendMail(mailOptions);
// Send the second email only if the first email is successful
await transporter.sendMail(mailOptions2);
return res.status(200).json({
message: "Thank you for contacting us!",
});
} catch (error) {
console.error(error);
return res.status(500).send({
msgType: "error",
message: "Check fields.....",
});
}
} else {
return res.status(405).send({
msgType: "error",
message: "Method not allowed",
});
}
}
Also Read : You may need a contact form, here’s the code!
Wrapping Up
In this tutorial, we’ve explored the integration of Nodemailer into a Next.js project, creating a robust contact form with the capability to send informative emails. Feel free to adapt and enhance this code to fit your application’s needs.
That’s it! You now possess the knowledge to integrate Nodemailer seamlessly into your Next.js application. If you have any questions or want to further customize the implementation, feel free to explore Nodemailer’s documentation for additional features and configurations. Happy coding!