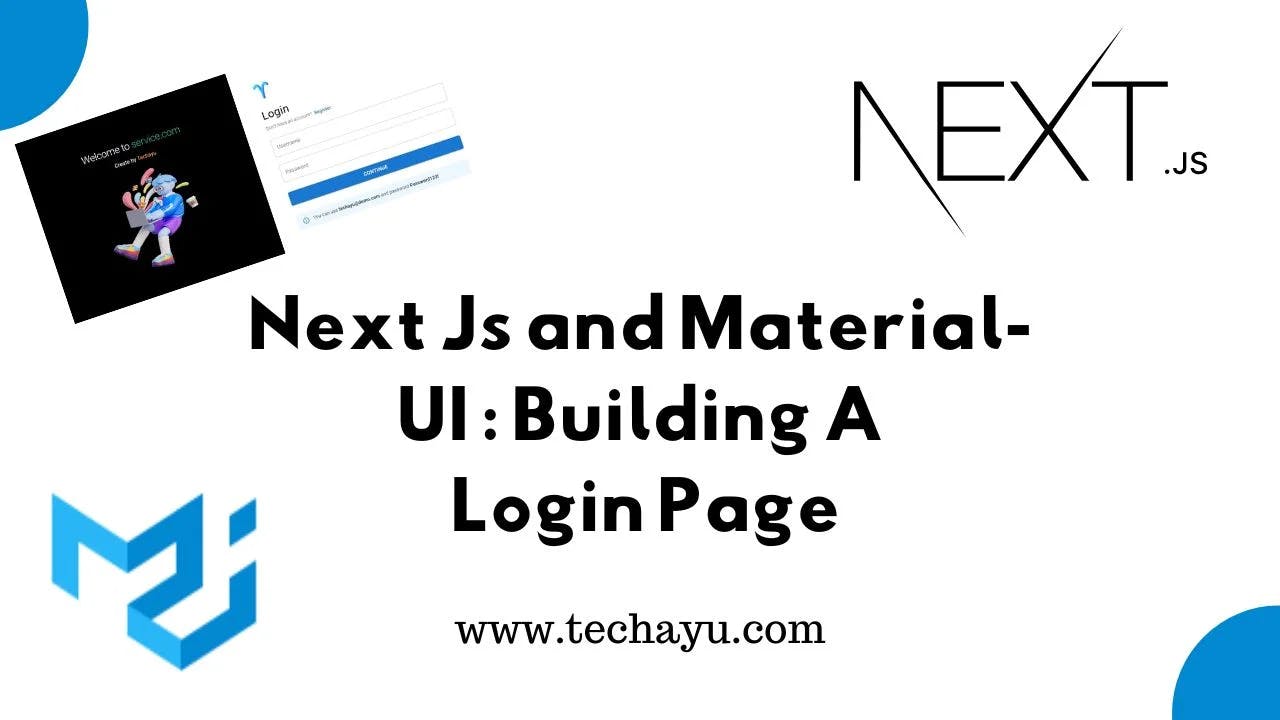
Next.Js And Material UI: Building A Login Page
React-Next JS and Material-UI Harmony: Designing a Secure and Stylish Sign-In Experience
Unlock the power of Next.js and Material-UI in a step-by-step guide. Elevate your sign-in experience with seamless design, web security, and form validation—creating a stylish, secure, and user-friendly authentication process.
Also Read : Crafting an Interactive Contact Form with Material-UI in React.
A Glimpse into the Code
Let’s dissect the key elements of the Sign-In page code:
Form Validation with Formik and Yup
The useFormik
hook from Formik and Yup are employed for form state management and validation.
Stylish UI with Material-UI
Material-UI components such as TextField
, Button
, Typography
, and Alert
are used to create an aesthetically pleasing and user-friendly sign-in form.
Handling Form Submission with Axios
The onSubmit
function of the Formik hook is responsible for handling form submissions. In this case, Axios is utilized to make a POST request to a specified API endpoint (/api/Signin
).
User Interface Split with Next.js Image Component
The Sign-In page is visually split into two sections, with the left side showcasing the service logo and a welcome message. The right side features the sign-in form.
Customizing the Sign-In Page
This Sign-In page is highly customizable for various projects. You can tweak the styling, add branding elements, or integrate it seamlessly into your existing Next.js application.
// pages/index.js
import Head from "next/head";
import NextLink from "next/link";
import { useRouter } from "next/router";
import { useFormik } from "formik";
import * as Yup from "yup";
import {
Alert,
Box,
Button,
Link,
Stack,
TextField,
Typography,
Grid,
} from "@mui/material";
import axios from "axios";
import Image from "next/image";
const Index = () => {
const router = useRouter();
const validationSchema = Yup.object({
username: Yup.string().required("Username is required"),
password: Yup.string().required("Password is required"),
});
const formik = useFormik({
initialValues: {
username: "",
password: "",
},
validationSchema: validationSchema,
onSubmit: async (values) => {
let payload = {
username: values.username,
password: values.password,
};
try {
const response = await axios.post(`/api/Signin`, payload);
if (response.status === 200) {
router.push("/dashboard");
formik.resetForm();
}
} catch (error) {
console.log("Errors", error);
}
},
});
return (
<>
<Head>
<title>Sign-in Page</title>
</Head>
<Box component="main">
<Grid container>
<Grid
item
xs={12}
lg={6}
sx={{
alignItems: "center",
background: "#000000",
color: "#ffffff",
display: "flex",
justifyContent: "center",
}}
>
<Box
sx={{
p: 4,
}}
>
<Typography
align="center"
color="inherit"
sx={{
fontSize: 30,
lineHeight: "32px",
mb: 2,
}}
variant="h1"
>
Welcome to{" "}
<Box component="a" sx={{ color: "#15B79E" }} target="_blank">
service.com
</Box>
</Typography>
<Typography align="center" sx={{ mb: 3 }} variant="subtitle1">
Create by <span style={{ color: "orange" }}>Techayu</span>
</Typography>
<Image alt="tech" src="/tech.png" width={300} height={300} />
</Box>
</Grid>
<Grid item xs={12} lg={6}>
<Box
sx={{
flex: "1 1 auto",
alignItems: "center",
display: "flex",
justifyContent: "center",
}}
>
<Box
sx={{
maxWidth: 550,
px: 3,
py: 6,
width: "100%",
}}
>
<div>
<Stack spacing={1} sx={{ mb: 3 }}>
<Box
sx={{
alignItems: "center",
py: 2,
}}
>
<Image
alt="logo"
src="/logo.png"
width={50}
height={50}
/>
</Box>
<Typography variant="h4">Login</Typography>
<Typography color="text.secondary" variant="body2">
Don't have an account?
<Link
component={NextLink}
href="/"
underline="hover"
variant="subtitle2"
>
Register
</Link>
</Typography>
</Stack>
<form onSubmit={formik.handleSubmit}>
<Stack spacing={3}>
<TextField
fullWidth
label="Username"
name="username"
type="text"
value={formik.values.username}
onChange={formik.handleChange}
error={
formik.touched.username &&
Boolean(formik.errors.username)
}
helperText={
formik.touched.username && formik.errors.username
}
/>
<TextField
fullWidth
label="Password"
name="password"
type="password"
value={formik.values.password}
onChange={formik.handleChange}
error={
formik.touched.password &&
Boolean(formik.errors.password)
}
helperText={
formik.touched.password && formik.errors.password
}
/>
</Stack>
<Button
fullWidth
size="large"
sx={{ mt: 4 }}
type="submit"
variant="contained"
>
Continue
</Button>
<Alert color="primary" severity="info" sx={{ my: 4 }}>
<div>
You can use <b>techayu@demo.com</b> and password{" "}
<b>Password123!</b>
</div>
</Alert>
</form>
</div>
</Box>
</Box>
</Grid>
</Grid>
</Box>
</>
);
};
export default Index;
Conclusion
In conclusion, the Sign-In page serves as the first interaction point for users, making it a critical component in any web application. Understanding the intricacies of its implementation with Next.js, Material-UI, and Formik can significantly enhance user experience and security.
All Related Posts
- MUI Table Integration, Pagination, & Excel Export
- Cloudinary Setup In Next.Js: A Step-By-Step Guide
- How To Secure APIs In Next JS
- How to use Nodemailer in Next JS
- How To Use MUI Grid For Responsive Web Layouts
- How To Create A Multiform In MUI And Next Js?
- Material-UI Typography: React Text Styling Guide
- How To Create Contact Form With MUI In React
- How To Use Material UI Forms
- MUI AppBar For Top-Level Navigation In React
- MUI Card Components: Elevate Your React UI Design
- How To Use Material-UI Cards