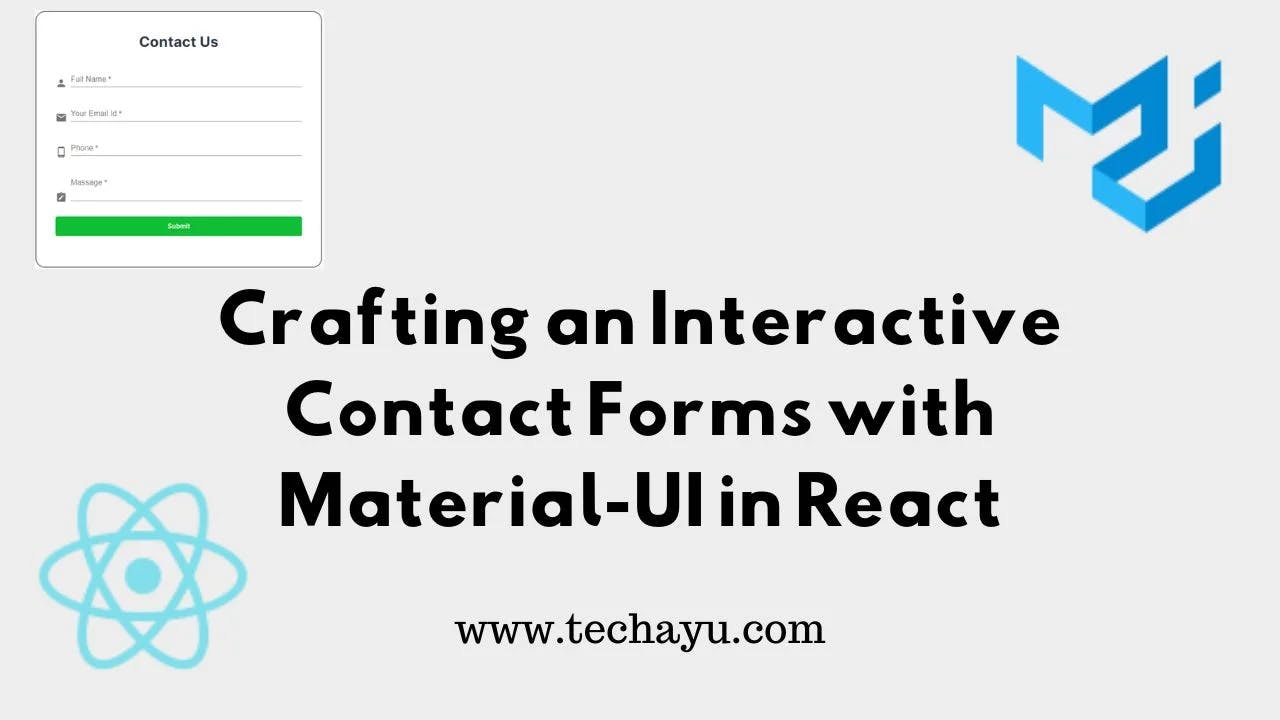
How To Create Contact Form With MUI In React
Streamlining User Communication: Crafting an Interactive Contact Form with Material-UI in React
Empower your React application with a user-friendly and visually appealing contact form using Material-UI components. In this comprehensive guide, we’ll walk through the process of creating a dynamic and responsive contact form that not only enhances user experience but also integrates seamlessly into your project.
Integrating Material-UI Components
Begin by importing essential Material-UI components such as Button
, TextField
, Box
, Typography
, and icons like PersonIcon
, EmailIcon
, NoteAltIcon
, and PhoneAndroidIcon
. This establishes a solid foundation for the form’s structure and styling.
// (Import statements)
import React, { useState } from "react";
import { Button, TextField, Box, Typography } from "@mui/material";
import PersonIcon from "@mui/icons-material/Person";
import EmailIcon from "@mui/icons-material/Email";
import NoteAltIcon from "@mui/icons-material/NoteAlt";
import PhoneAndroidIcon from "@mui/icons-material/PhoneAndroid";
import axios from "axios";
const ContactForm = () => {
// State for form data
const [formData, setFormData] = useState({
fullname: "",
email: "",
phone: "",
msg: "",
});
const { fullname, email, phone, msg } = formData;
// ... (handleChange and handleSubmit functions)
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = async (e) => {
e.preventDefault();
const payload = {
fullname: fullname,
email: email,
phone: phone,
msg: msg,
};
try {
const response = await axios.post(`/api/contact`, payload, {
headers: {
"Content-Type": "application/json",
},
});
if (response.status === 200) {
alert(response.data.message);
setFormData({
fullname: "",
email: "",
phone: "",
msg: "",
});
}
} catch (error) {
if (error.response && error.response.status === 400) {
alert(error.response.data.message);
}
if (error.response && error.response.status === 404) {
alert(error.response.data.message);
}
if (error.response && error.response.status === 500) {
alert(error.response.data.message);
}
}
};
// JSX structure for the contact form
return (
<>
<Box
sx={{ alignItems: "center", justifyContent: "center", display: "flex" }}
>
<Box
sx={{
background: "#ffffff",
width: 600,
border: "2px solid #767676 ",
borderRadius: 5,
p: 5,
my: 2,
mx: 2,
}}
>
<Typography
sx={{
color: "#2D3748",
fontFamily: " 'Inter', sans-serif",
fontSize: 30,
fontWeight: 700,
}}
textAlign="center"
>
Contact Us
</Typography>
<Box
component="form"
validate="true"
onSubmit={handleSubmit}
sx={{ mt: 1 }}
>
<Box sx={{ display: "flex", alignItems: "flex-end" }}>
<PersonIcon sx={{ color: "action.active", mr: 1, my: 0.5 }} />
<TextField
variant="standard"
margin="normal"
required
fullWidth
onChange={handleChange}
value={fullname}
name="fullname"
id="fullname"
label="Full Name"
/>
</Box>
<Box sx={{ display: "flex", alignItems: "flex-end" }}>
<EmailIcon sx={{ color: "action.active", mr: 1, my: 0.5 }} />
<TextField
variant="standard"
margin="normal"
required
fullWidth
onChange={handleChange}
value={email}
name="email"
id="email"
autoComplete="email"
label="Your Email Id"
/>
</Box>
<Box sx={{ display: "flex", alignItems: "flex-end" }}>
<PhoneAndroidIcon
sx={{ color: "action.active", mr: 1, my: 0.5 }}
/>
<TextField
variant="standard"
margin="normal"
required
fullWidth
onChange={handleChange}
value={phone}
name="phone"
id="phone"
label="Phone"
/>
</Box>
<Box sx={{ display: "flex", alignItems: "flex-end" }}>
<NoteAltIcon sx={{ color: "action.active", mr: 1, my: 0.5 }} />
<TextField
variant="standard"
margin="normal"
required
fullWidth
onChange={handleChange}
value={msg}
name="msg"
id="msg"
label="Massage"
multiline
rows={2}
/>
</Box>
<Button
type="submit"
fullWidth
sx={{
my: 3,
fontFamily: " 'Inter', sans-serif",
letterSpacing: "normal",
py: 1,
color: "#fff",
backgroundColor: "#10BE35 ",
"&:hover": { backgroundColor: "#07711E" },
textTransform: "none",
}}
>
Submit
</Button>
</Box>
</Box>
</Box>
</>
);
};
export default ContactForm;
Enhancing User Experience
- Dynamic Form Handling: Utilize React’s state management to handle form data dynamically, creating a seamless user experience.
- Axios for API Interaction: Employ Axios to handle form submissions, interacting with the backend API endpoint for smooth data transmission.
- Styling with Material-UI: Leverage Material-UI’s styling capabilities to create an aesthetically pleasing and responsive contact form.
Conclusion: Elevate User Interaction with Material-UI Contact Form
Integrate this Material-UI contact form into your React project to establish effective communication channels with users. Elevate user interaction, capture essential data, and create a visually appealing contact experience.
Feel free to customize the code to align with your project’s design requirements and explore additional Material-UI features for further enhancement.
By combining Material-UI components, state management, and API interaction, you’ll create a contact form that seamlessly integrates into your React application, ensuring a positive user experience.
All Related Posts
- MUI Table Integration, Pagination, & Excel Export
- How To Use MUI Grid For Responsive Web Layouts
- How To Create A Multiform In MUI And Next Js?
- Material-UI Typography: React Text Styling Guide
- Next.Js And Material UI: Building A Login Page
- How To Use Material UI Forms
- MUI AppBar For Top-Level Navigation In React
- MUI Card Components: Elevate Your React UI Design
- How To Use Material-UI Cards
- How To Use React.Js PropTypes
- How to Implement Custom Validation in React JS
- How To Use Axios In React Js